实现了 相机边界 & 跟随Actor & 跟随死区 的功能
效果
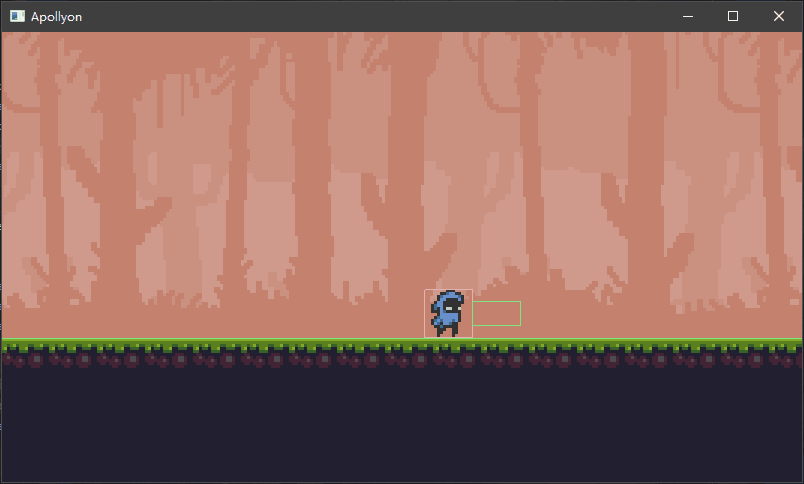
代码
GameConstant.PIXEL_PER_UNIT
为项目自定义数值, 此处为16
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92
| import com.badlogic.gdx.graphics.OrthographicCamera; import com.badlogic.gdx.math.Rectangle; import com.badlogic.gdx.math.Vector2; import com.badlogic.gdx.math.Vector3; import com.badlogic.gdx.scenes.scene2d.Actor; import io.tomoto.apollyon.utils.GameConstant; import lombok.Getter; import lombok.Setter;
@Getter @Setter public class FancyCamera extends OrthographicCamera {
private Rectangle boundary;
private Vector2 deadZone;
private Actor target;
private boolean lerp;
public FancyCamera() { deadZone = new Vector2(0, 0); }
@Override public void update() { if (target == null) { return; }
Vector2 targetPosition = target.localToParentCoordinates(new Vector2(0, 0)); Vector3 finalPosition = new Vector3(position);
if (lerp) { if (position.x - deadZone.x > targetPosition.x || position.x + deadZone.x < targetPosition.x) { finalPosition.x = targetPosition.x; } if (position.y - deadZone.y > targetPosition.y || position.y + deadZone.y < targetPosition.y) { finalPosition.y = targetPosition.y; } }
if (boundary != null) { if (finalPosition.x < boundary.x) { finalPosition.x = boundary.x; } if (finalPosition.y < boundary.y) { finalPosition.y = boundary.y; } if (finalPosition.x > boundary.x + boundary.width) { finalPosition.x = boundary.x + boundary.width; } if (finalPosition.y > boundary.y + boundary.height) { finalPosition.y = boundary.y + boundary.height; } }
if (lerp) { finalPosition.x = position.x + (finalPosition.x - position.x) / (GameConstant.PIXEL_PER_UNIT * 2); finalPosition.y = position.y + (finalPosition.y - position.y) / (GameConstant.PIXEL_PER_UNIT * 2); }
position.set(finalPosition); super.update(); } }
|
用例
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Override public void show() { this.camera = new FancyCamera(); this.camera.position.set(viewport.getWorldWidth() / 2, viewport.getWorldHeight() / 2, 0);
this.camera.setTarget(this.stage.getApollyon()); this.camera.setLerp(true); this.camera.setBoundary(new Rectangle( viewport.getWorldWidth() / 2, viewport.getWorldHeight() / 2, stage.getMapWidth() - viewport.getWorldWidth(), stage.getMapHeight() - viewport.getWorldHeight())); this.camera.setDeadZone(new Vector2(Player.SIZE / GameConstant.PIXEL_PER_UNIT, 0)); }
|